Source: How-To Geek
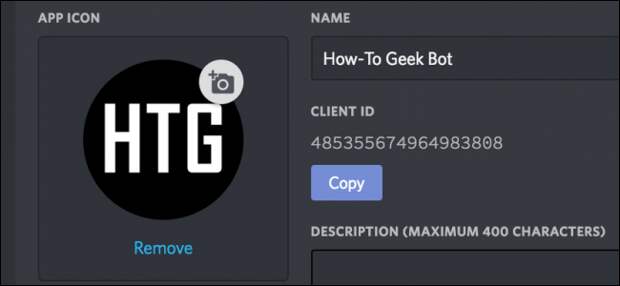
Discord has an excellent API for writing custom bots, and a very active bot community. Today we’ll take a look at how to get started making your own.
You will need a bit of programming knowledge to code a bot, so it isn’t for everyone, but luckily there are some modules for popular languages that make it very easy to do.
We’ll be using the most popular one, discord.js.Getting Started
Head over to Discord’s bot portal, and create a new application.
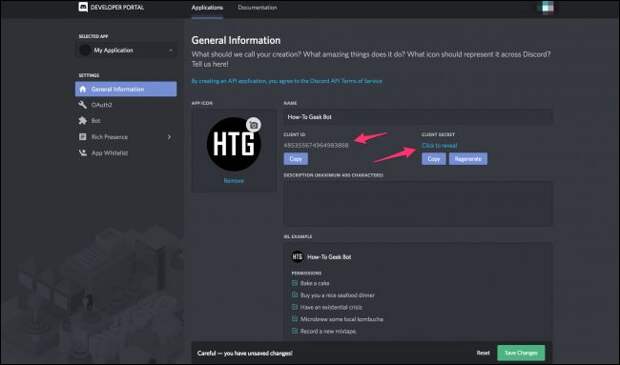
You’ll want to make a note of the Client ID and secret (which you should keep a secret, of course). However, this isn’t the bot, just the “Application.” You’ll have to add the bot under the “Bot” tab.

Make a note of this token as well, and keep it a secret. Do not, under any circumstances, commit this key to Github. Your bot will be hacked almost immediately.
Install Node.js and Get Coding
To run Javascript code outside of a webpage, you need Node. Download it, install it, and make sure it works in a terminal (or Command Prompt, as all of this should work on Windows systems). The default command is “node.”
We also recommend installing the nodemon tool. It’s a command line app that monitors your bot’s code and restarts automatically on changes. You can install it by running the following command:
npm i -g nodemon
You’ll need a text editor. You could just use notepad, but we recommend either Atom or VSC.
Here’s our “Hello World”:

const Discord = require('discord.js'); const client = new Discord.Client(); client.on('ready', () => { console.log(`Logged in as ${client.user.tag}!`); }); client.on('message', msg => { if (msg.content === 'ping') { msg.reply('pong'); } }); client.login('token');
This code is taken from the discord.js example. Let’s break it down.
- The first two lines are to configure the client. Line one imports the module into an object called “Discord,” and line two initializes the client object.
- The
client.on('ready')
block will fire when the bot starts up. Here, it’s…
The post How to Make Your Own Discord Bot appeared first on FeedBox.